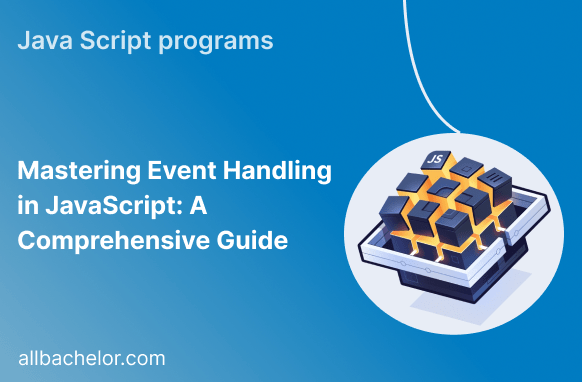
Introduction
Interactivity is the heart and soul of web applications. JavaScript, with its ability to respond to user actions, plays a pivotal role in creating dynamic and engaging web experiences. In this comprehensive guide, we will explore the world of event handling in JavaScript, equipping you with the knowledge and practical examples needed to build interactive and responsive web applications.
Table of Contents
Understanding Events
Event Handlers and Listeners
Common DOM Events
Event Object
Event Bubbling and Capturing
Preventing Default Behavior
Event Delegation
Custom Events
Asynchronous Event Handling
Conclusion
1. Understanding Events
Events are actions or occurrences that happen in the browser, such as a button click, mouse movement, or keyboard input. JavaScript allows you to capture and respond to these events, making your web applications interactive.
2. Event Handlers and Listeners
Event handling in JavaScript can be done using event handlers (e.g., onclick
, onmouseover
) or event listeners. Event listeners are preferred because they provide better separation of concerns and allow multiple functions to respond to the same event.
// Using an event listener
element.addEventListener("click", function() {
// Handle the click event
});
3. Common DOM Events
There is a wide range of DOM events you can listen for, including click, mouseover, keyup, submit, and more. Understanding when and how to use these events is key to building responsive user interfaces.
// Example: Listening for a button click
button.addEventListener("click", function() {
// Button clicked!
});
4. Event Object
When an event occurs, JavaScript passes an event object containing information about the event to the event handler. This object includes properties like event.type
, event.target
, and event.preventDefault()
.
element.addEventListener("click", function(event) {
console.log(event.type); // Click
console.log(event.target); // The clicked element
});
5. Event Bubbling and Capturing
Events in the DOM follow a bubbling and capturing phase. Understanding this propagation model is crucial when dealing with nested elements and event delegation.
6. Preventing Default Behavior
Certain events, like form submissions and anchor clicks, have default browser behaviors. You can prevent these behaviors using event.preventDefault()
to handle these actions in JavaScript.
// Preventing form submission
form.addEventListener("submit", function(event) {
event.preventDefault(); // Prevent the form from submitting
// Custom form handling logic
});
7. Event Delegation
Event delegation is a technique where you attach a single event listener to a common ancestor of multiple elements. It’s useful for efficiently handling events on dynamically generated elements or a list of items.
// Event delegation example
list.addEventListener("click", function(event) {
if (event.target.tagName === "LI") {
// Handle the click on an LI element
}
});
8. Custom Events
In addition to standard DOM events, you can create custom events to add interactivity to your web applications. Custom events can be dispatched and listened to like native events.
// Creating and dispatching a custom event
let customEvent = new Event("customEvent");
element.dispatchEvent(customEvent);
// Listening for the custom event
element.addEventListener("customEvent", function() {
// Handle the custom event
});
9. Asynchronous Event Handling
Some events may involve asynchronous operations, such as making API requests. Understanding how to handle asynchronous events and manage their callbacks is crucial for responsive applications.
10. Conclusion
Event handling is the backbone of interactive web development. With JavaScript’s power and flexibility, you can create web applications that respond to user actions, update content dynamically, and provide a seamless user experience.
As you continue your journey into web development, explore advanced topics like event propagation, handling touch and mobile events, and working with third-party libraries like jQuery for event handling. The possibilities are endless, and your skills in event handling are the key to unlocking them.
Stay tuned for more articles that delve deeper into the world of JavaScript and web development. Happy coding!
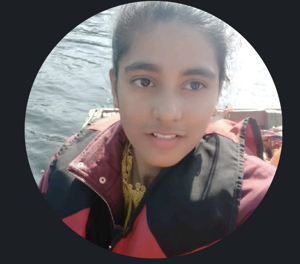
Write a comment ...